I woke up this morning thinking about Armageddon and how fragile we are as a planet if an asteroid comes our way. I am also intrigued by exploring space and the technologies available from my desk. I will show you how to combine two APIs to be alerted via SMS when an asteroid is passing nearby (relatively speaking).
NASA OpenAPI
The first resource is NASA’s open API portal. You could use tons of great interfaces for your ideas, but the one that interests me, in this case, is NeoWs (Near Earth Object Web Service).
Unlock
After reading about the API, the first step in your journey is to obtain an API key. This is done by filling your details into a form and providing a valid e-mail.
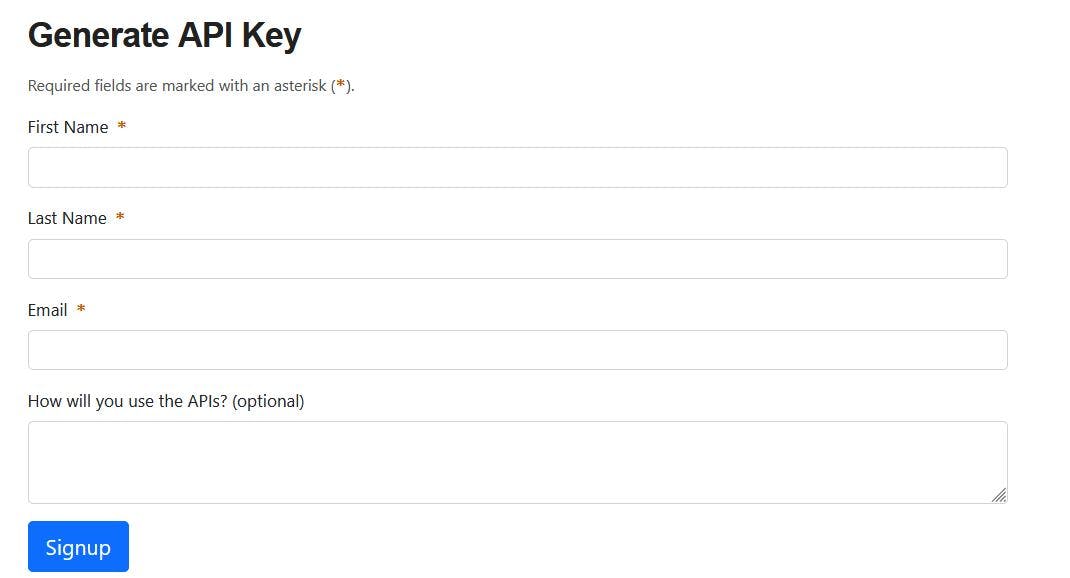
A screen showing the NASA API registration form
Please note that most APIs have an API limit of 1000 requests per hour. They are suitable for tests and personal benefit but not for production-ready usage
Explore
Let’s spend a few minutes to see the data returned by the API to build our use case.
The request (GET)
- start_date (YYYY-MM-DD) – Starting date for asteroid search
- end_date (YYYY-MM-DD) – Ending date for asteroid search
- api_key – the key you received via e-mail after the previous step.
The response
It returns a JSON object with valuable data, which we must work with to get what we need. Look at the data here.
We have a structure called near_earth_objects which contains the details we need in a complex structure:
- estimated_diameter – the diameter of the asteroid in meters, kilometers, miles, and feet.
- relative_velocity – the relative velocity of the object
- miss_distance – the distance away from the orbiting_body
- orbiting_body – in most cases, is Earth, but you could explore more options if you like.
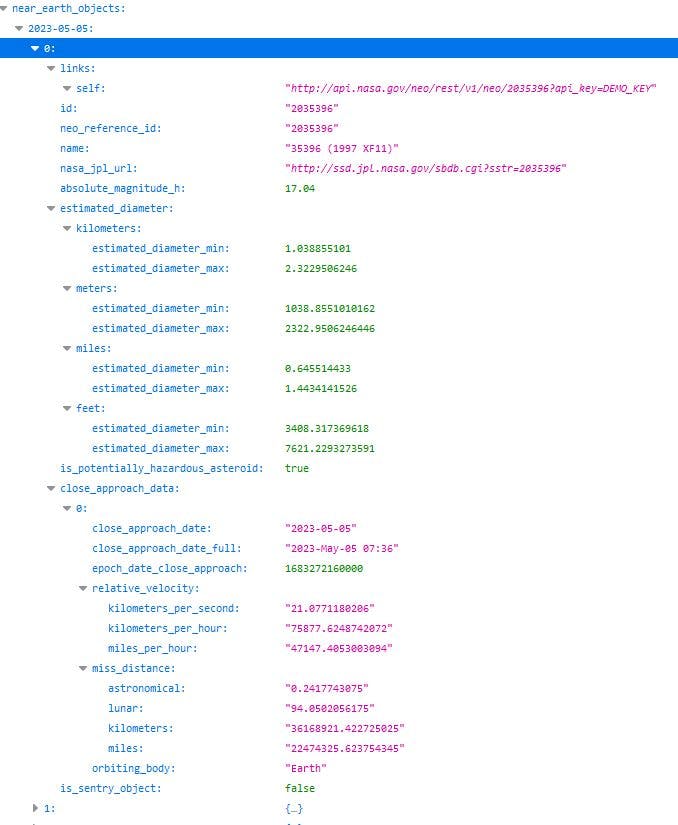
A screen showing the JSon object received as a response to the request above.
Run
So we have all the objects passing nearby and want to get the closest one and alert us daily. Let’s use your Python skills to do that.
#Settings and URL to conect to NASA API
#Get your free API key from here: https://api.nasa.gov/
ad_today = date.today().strftime("%Y-%m-%d")
url = "https://api.nasa.gov/neo/rest/v1/feed?start_date="+ad_today+"&end_date="+ad_today+"&api_key=[your keu]"
#Hadle the responce json
response = requests.request("GET", url)
response.encoding = 'utf-8'
jsn = response.json()
if "near_earth_objects" in jsn:
base = jsn['near_earth_objects'][ad_today]
i = findClosestEncounter(base)
#extract the data we need to create the alert
name = base[i]['name']
to_appear = base[i]['close_approach_data'][0]['close_approach_date_full']
how_close = base[i]['close_approach_data'][0]['miss_distance']['kilometers']
dia_meter = base[i]['estimated_diameter']['meters']['estimated_diameter_max']
The findClosestEncounter function helps you find the closest object to Earth from the bucket of all things passing nearby. Maybe there is a more elegant solution, but this one works for me well.
def findClosestEncounter(jd):
# a simple function for discovering the nearest object for the day from all registered objects
asteroids = []
for i in range(0, len(jd)):
asteroids.insert(i,jd[i]['close_approach_data'][0]['miss_distance']['kilometers'])
return asteroids.index(min(asteroids))
Make it human-readable.
Since we will send an SMS, formatting the data is a good idea. Feel free to use another formatting as well.
#format the data
howclose = round(float(how_close))
diameter = round(dia_meter)
Build the message you want to send via SMS.
#build the message
alert ="The nearest asteroid for today is "+ name+". It will be "+str(howclose)+" km away with a diameter of "+str(diameter)+" meters."
NeoWs recap
What have you done so far?
- You have an API key for the NASA OpenAPI portal
- You explored the NeoWs API
- You extracted the closest object to Earth from all the objects that could pass nearby.
- You crafted a message to alert yourself to this encounter.
Let’s send that SMS.
There is another API I want to introduce in this short but helpful example – Messagebird.
Release the bird
Visit their website and register to get an API key
After the registration, you can send a few complimentary SMS messages delivered to your actual phone number. Let’s add this functionality to your Python code.
#SMS client
#Get your free API key from here: https://developers.messagebird.com/api/#api-endpoint
sms = messagebird.Client("your API key here")
#Prepare and send the message to a phone number of your choice.
# Change the name "Asteroid" to something you want. It will appear as a sender
message = sms.message_create(
'Asteroid',
'+yourphonenumner',
alert,
{ 'reference' : 'Asteroid' }
)
Get the Armageddon Alert
You can find the complete script here.
Put all the pieces together and run your code to see whether you did well. Now you can sleep better, knowing you will be alerted about every big object flying towards the Earth. Of course, NASA needs to detect it first.
If you like the result, you could put it in a cron job and trigger it once a day.
Happy Hacking!
This article was originally published by Bogomil Shopov – Бого on Hackernoon.